1. 什么是UGF
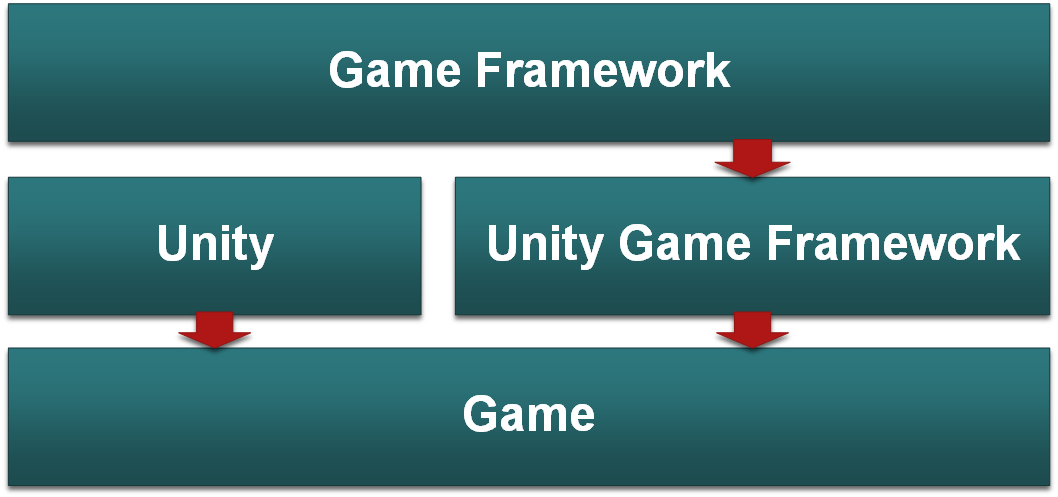
2. 安装UGF包
在下载页面下载最新版 Game Framework(说好不用 git 就不用),当前使用的版本是 2020.07.10,下载后,可以获得一个叫做 GameFramework_2020_07_10.unitypackage 的 Unity 插件包。

建议时刻考虑使用较新版本的 Game Framework,较新的版本出了新增特性和修正一些 BUG 之外,还会不断地优化框架自身逻辑的内存开销。
将插件的全部内容导入刚创建的工程。
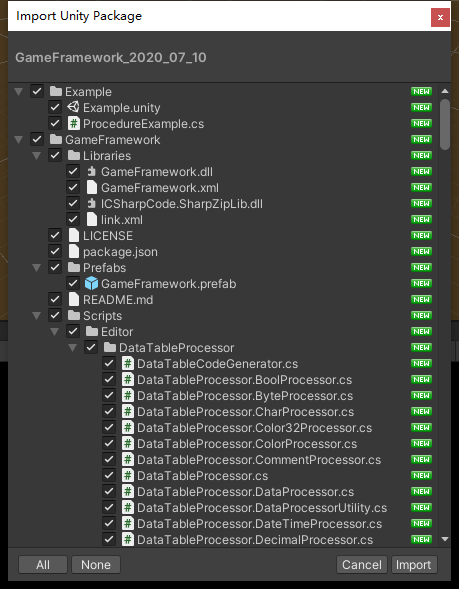
3. 工程结构
GameFramework 文件夹是框架的全部内容,其中:
- Libraries
存放 GameFramework.dll 核心框架和一些框架必需的第三方库(当前只有一个开源 zip 压缩算法库)
- Prefabs
存放 GameFramework.prefab 预制体,用于快速创建一个游戏框架启动场景
- Scripts
存放 UnityGameFramework 的全部 Runtime 和 Editor 代码
Example 文件夹是一个示例目录,其中:
- Example.unity
是一个含有 GameFramework.prefab 预制体的空场景,作为游戏启动的场景
- ProcedureExample.cs
是一个示例流程代码文件,示例将以这个流程作为启动流程。
打开 Example/Example.unity 场景,此时 Hierarchy 窗口显示了 Game Framework 的各个组成组件。
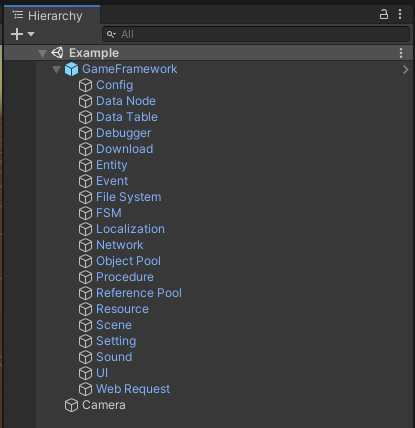
4. 组件的获取
在 Game Framework 中,获取一个内置组件的代码是这样的:
1 2 3 4
| BaseComponent baseComponent = UnityGameFramework.Runtime.GameEntry.GetComponent<BaseComponent>();
baseComponent.EditorResourceMode = true;
|
每次这么用,有点繁琐。一般把 Game Framework 的组件封装为能够全局静态访问的属性。
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209
| namespace UnityGameFramework.Runtime { public partial class GameEntry { public static BaseComponent Base { get; private set; }
public static ConfigComponent Config { get; private set; }
public static DataNodeComponent DataNode { get; private set; }
public static DataTableComponent DataTable { get; private set; }
public static DebuggerComponent Debugger { get; private set; }
public static DownloadComponent Download { get; private set; }
public static EntityComponent Entity { get; private set; }
public static EventComponent Event { get; private set; }
public static FileSystemComponent FileSystem { get; private set; }
public static FsmComponent Fsm { get; private set; }
public static LocalizationComponent Localization { get; private set; }
public static NetworkComponent Network { get; private set; }
public static ObjectPoolComponent ObjectPool { get; private set; }
public static ProcedureComponent Procedure { get; private set; }
public static ResourceComponent Resource { get; private set; }
public static SceneComponent Scene { get; private set; }
public static SettingComponent Setting { get; private set; }
public static SoundComponent Sound { get; private set; }
public static UIComponent UI { get; private set; }
public static WebRequestComponent WebRequest { get; private set; }
public static void InitBuiltinComponents() { Base = GameEntry.GetComponent<BaseComponent>(); Config = GameEntry.GetComponent<ConfigComponent>(); DataNode = GameEntry.GetComponent<DataNodeComponent>(); DataTable = GameEntry.GetComponent<DataTableComponent>(); Debugger = GameEntry.GetComponent<DebuggerComponent>(); Download = GameEntry.GetComponent<DownloadComponent>(); Entity = GameEntry.GetComponent<EntityComponent>(); Event = GameEntry.GetComponent<EventComponent>(); FileSystem = GameEntry.GetComponent<FileSystemComponent>(); Fsm = GameEntry.GetComponent<FsmComponent>(); Localization = GameEntry.GetComponent<LocalizationComponent>(); Network = GameEntry.GetComponent<NetworkComponent>(); ObjectPool = GameEntry.GetComponent<ObjectPoolComponent>(); Procedure = GameEntry.GetComponent<ProcedureComponent>(); Resource = GameEntry.GetComponent<ResourceComponent>(); Scene = GameEntry.GetComponent<SceneComponent>(); Setting = GameEntry.GetComponent<SettingComponent>(); Sound = GameEntry.GetComponent<SoundComponent>(); UI = GameEntry.GetComponent<UIComponent>(); WebRequest = GameEntry.GetComponent<WebRequestComponent>(); } } }
|
将其放到Assets/GameFramework/Scripts/Runtime/Base/GameEntryInit.cs路径下即可。